As a developer, I’ve always been fascinated by the flexibility and power of microservices architecture. This approach breaks down a monolithic application into multiple small, independent services, each focusing on a specific task. In this article, I will share some of my experiences and insights, guiding you through what microservices are and how microservices work.
1. Container Technology: The Foundation of Microservices
When I first delved into microservices, container technology was the initial area I explored in depth. Containerization technologies like Docker fundamentally changed my perspective on software deployment. Docker allows us to package applications and their dependencies into portable container images, ensuring consistency across different environments.
- Docker: This is perhaps the tool I use the most. It simplifies deployment, supports rapid container startup and shutdown, and significantly boosts development and deployment efficiency. With Docker, I can easily create and destroy development environments, dramatically speeding up development and testing cycles. Docker’s container images ensure consistency across environments, solving the “it works on my machine” problem.
- Podman: Similar to Docker but without the daemon, making it my choice for projects needing finer control. Podman allows me to run containers as a non-root user, enhancing system security.
- LXC (Linux Containers): An early container technology offering more granular control and isolation. LXC lets me create lightweight virtual environments, perfect for highly customized systems.
These container technologies ensure application consistency and facilitate rapid deployment and scaling, which are crucial for the success of microservices architecture. Containers not only simplify environment management but also significantly improve resource utilization and system elasticity.
2. Databases: Diversified Data Storage
In my projects, I’ve found that selecting the right database is critical for understanding how microservices work effectively. Choosing between SQL and NoSQL databases based on each service’s specific needs can greatly enhance data processing efficiency and flexibility.
- SQL Databases: Such as Postgres, Oracle, and MySQL, are ideal for structured data and complex queries.
- Postgres: I particularly favor Postgres for its powerful features and rich data types. Its extensibility and support for complex queries make it an excellent choice for financial systems and reporting.
- Oracle: In enterprise applications, Oracle’s high availability and reliability are irreplaceable. Its comprehensive features and robust performance optimization are widely used in critical business systems like banking and telecommunications.
- MySQL: My go-to database for web applications, MySQL is popular for its performance and ease of use. Its community edition and rich plugins make it widely used in small to medium-sized applications.
- NoSQL Databases: Such as DynamoDB, MongoDB, Cassandra, and HBase, are suitable for unstructured data and high scalability needs.
- DynamoDB: I’ve found DynamoDB’s auto-scaling feature particularly useful for handling large-scale data. Its serverless architecture and high availability make it ideal for e-commerce and IoT applications.
- MongoDB: Its flexible document storage makes rapid development and iteration easier. MongoDB’s schema-free structure is perfect for applications that need to quickly adapt to changes.
- Cassandra: I rely on Cassandra for real-time big data analytics projects to handle high-throughput write operations. Its decentralized architecture and linear scalability are excellent for big data and real-time analytics systems.
- HBase: My first choice for real-time read and write of large-scale data. HBase’s seamless integration with Hadoop makes it suitable for building big data storage and analytics platforms.
This diverse data storage strategy not only enhances system flexibility but also ensures that each service operates efficiently to meet different scenarios. By choosing the right database, I can ensure high data processing efficiency and system scalability.
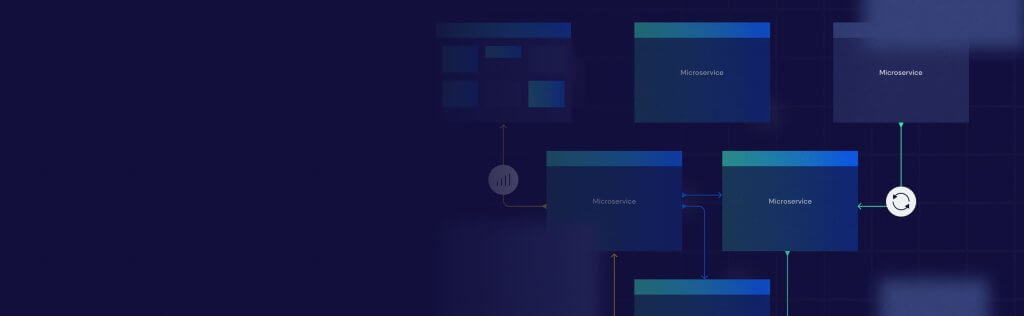
3. Security: Ensuring Secure Communication and Data
Understanding how microservices work involves ensuring secure communication and data handling. Frequent and distributed service communication requires robust security measures.
- JWT (JSON Web Token): Used for user authentication, ensuring every request is from an authenticated user. This standard is crucial when handling distributed systems. JWT enables easy implementation of single sign-on and distributed authentication, enhancing system security and user experience.
- TLS (Transport Layer Security): Ensures secure data transmission through encryption, preventing data theft and tampering. TLS encryption makes transmitting sensitive data over open networks safe and reliable.
With these security technologies, I can confidently develop and deploy microservices in a distributed environment without worrying about data breaches or unauthorized access. Security is the foundation of system stability and user trust, especially in applications dealing with financial and personal data.
4. Programming Languages: Diversity and Flexibility
In microservices architecture, I’ve found that choosing the right programming language is key to understanding how microservices work efficiently. Common languages include:
- .NET: Suitable for building high-performance enterprise applications. Its powerful development tools and extensive enterprise support make it highly efficient for developing complex business logic and high-performance applications.
- Golang: Its high concurrency handling and simple syntax make it one of my top choices for building microservices. Go’s high performance and ease of deployment make it perfect for building highly concurrent network services.
- Java: Java’s mature ecosystem is irreplaceable when building large-scale enterprise applications. Frameworks like Spring Boot make Java very convenient and efficient for developing microservices.
- Node.js: Based on JavaScript, it’s ideal for building high-performance web applications and real-time systems. Node.js’s asynchronous I/O and rich package management make it highly advantageous for building fast-responsive applications.
- Python: Its simple syntax and rich libraries make it my go-to for rapid development and prototyping. Python’s versatility and rich ecosystem make it popular in data science, automation scripts, and web development.
This diversity allows me to select the most suitable language for specific needs, enhancing development efficiency and system performance. Different programming languages have their strengths, and by choosing and combining them wisely, I can leverage each language’s advantages to build efficient microservices systems.
5. Cloud Providers: Elasticity and Scalability
Understanding how microservices work involves leveraging cloud providers to bring immense elasticity and scalability to microservices architecture. Common cloud providers include:
- GCP (Google Cloud Platform): Offers comprehensive cloud services, supporting high availability and low latency worldwide. GCP’s machine learning and big data processing services are advantageous for complex data analysis and AI applications.
- Azure: Integrated with Windows Server and SQL Server, making it suitable for enterprise applications. Azure’s hybrid cloud solutions and enterprise support make it widely used in enterprise IT environments.
- AWS (Amazon Web Services): My most frequently used cloud provider, offering a wide range of services and tools for various application needs. AWS’s extensive services and global coverage make it the go-to for building elastic and scalable applications.
Using these cloud providers, I can flexibly adjust resources, ensuring the system remains stable under high load. This flexibility and scalability allow me to quickly expand or reduce resources as needed, ensuring system efficiency and cost-effectiveness.
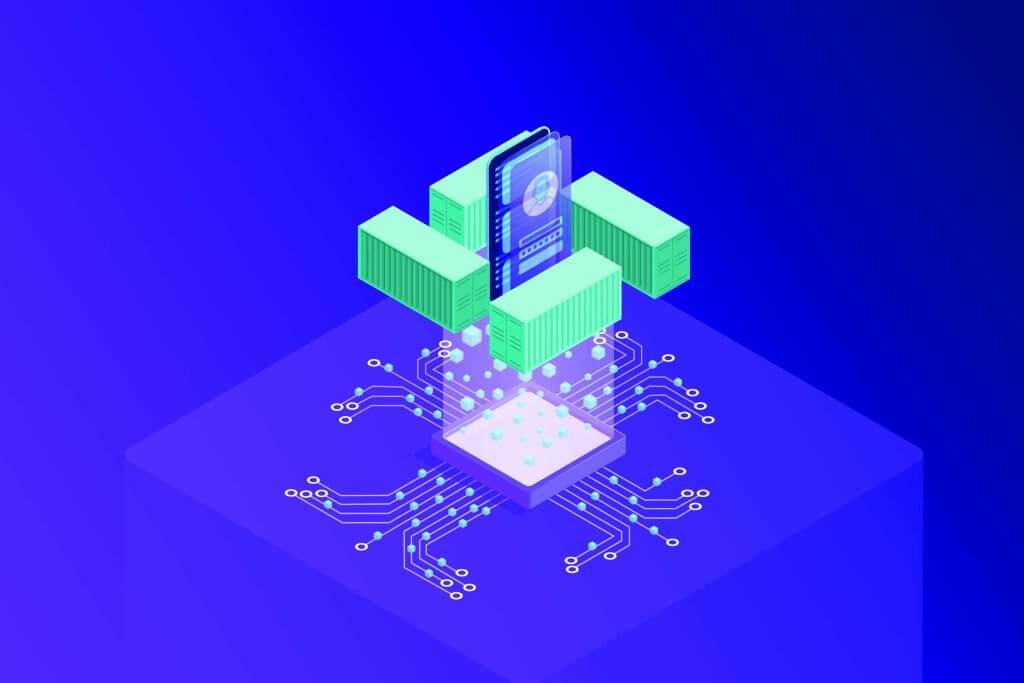
6. Caching: Accelerating Data Access
In high-frequency data access scenarios, caching technology is crucial to understanding how microservices work. Common caching tools include:
- Redis: Its various data structures and high performance make it my go-to for accelerating database queries and implementing distributed locks. Redis supports not only simple key-value storage but also complex data structures like lists, sets, and hashes, making it highly efficient for handling real-time data and high-frequency access.
- Hazelcast: A distributed in-memory data storage and computation platform, offering memory data grids and stream processing. Hazelcast’s in-memory data grid simplifies data sharing and quick access in distributed environments.
- Memcache: A high-performance distributed memory caching system, often used to speed up dynamic web applications. Memcache’s simplicity and high performance make it popular for web applications needing quick response times.
Using these caching tools, I can significantly improve system response speed and reduce database load, resulting in a smoother user experience. Caching not only speeds up data access but also enhances system scalability and stability.
7. Message Brokers: Decoupling and Asynchronous Communication
Message broker tools are key to understanding how microservices work by achieving asynchronous communication and decoupling between services. Common message broker tools include:
- Kafka: Its high throughput for real-time data stream processing makes it my first choice for log aggregation and real-time analysis. Kafka’s distributed architecture and high reliability are excellent for handling large-scale data streams.
- RabbitMQ: Supports multiple messaging protocols, offering flexible routing and message delivery mechanisms, suitable for building complex messaging systems. RabbitMQ’s rich features and scalable architecture make it highly effective in applications requiring complex message routing and processing.
Using these message broker tools, I can easily achieve asynchronous communication, ensuring loose coupling between services and enhancing system reliability and scalability. Message brokers not only decouple services but also ensure reliable message delivery and processing in distributed systems.
8. Load Balancers: High Availability and Performance Optimization
Load balancers are crucial for understanding how microservices work by ensuring even distribution of load across service instances and preventing single points of failure and performance bottlenecks. Common load balancers include:
- Nginx: A high-performance HTTP and reverse proxy server, supporting load balancing, caching, and WAF (Web Application Firewall) features. Nginx’s high performance and flexibility make it a top choice for web servers and reverse proxies.
- Traefik: A modern reverse proxy and load balancer, supporting automated service discovery and configuration, suitable for microservices and containerized environments. Traefik’s dynamic configuration and powerful integration capabilities make it very useful in environments needing quick response to changes.
- Seesaw: A high-performance load balancer based on Go, designed for internal use by Google, offering high performance and reliability. Seesaw’s high performance and flexible configuration make it effective in applications requiring high throughput and low latency.
Using load balancers, I can ensure system stability under high load and dynamically adjust resources based on traffic conditions, enhancing system elasticity and performance. Load balancing not only improves system availability but also optimizes performance and user experience.
9. Monitoring and Alerting: Ensuring System Health
Real-time monitoring and alerting are crucial for understanding how microservices work by ensuring system health. The tools I commonly use include:
- Kibana: An open-source analytics and visualization platform integrated with Elasticsearch, providing real-time data search, viewing, and interaction capabilities. Kibana’s powerful visualization features make monitoring data intuitive and easy to understand.
- Prometheus: An open-source system monitoring and alerting toolkit, suitable for recording real-time metrics data, offering flexible query and alerting functions. Prometheus’s efficient data storage and flexible query language make it very effective for monitoring large-scale distributed systems.
- Grafana: An open-source metrics analysis and visualization tool, integrated with multiple data sources, supporting dynamic and interactive dashboards. Grafana’s flexibility and powerful integration capabilities make it ideal for building monitoring and alerting systems.
Using these tools, I can monitor the system’s running status in real-time, quickly locate and resolve potential issues, ensuring high availability and performance. Monitoring and alerting not only improve system stability but also help prevent and quickly respond to failures.
10. API Gateways: Unified Entry and Management
API gateways are essential for understanding how microservices work by providing a unified entry point, managing and routing all API requests, and simplifying client-to-service communication. Common API gateways include:
- MuleSoft: An integration platform offering comprehensive API management and integration solutions, suitable for complex enterprise applications. MuleSoft’s powerful features and flexible integration capabilities make it highly effective in building and managing complex APIs.
- Ocelot: An open-source API gateway based on .NET, designed for microservices architecture, offering routing, authentication, rate limiting, and other functions. Ocelot’s simple configuration and powerful features make it very efficient in microservices projects in the .NET environment.
- Kong: An open-source API gateway supporting high-performance, scalable API management with a rich plugin system. Kong’s high performance and scalability make it effective in systems needing to handle large numbers of API requests.
Using API gateways, I can simplify microservices management and maintenance while achieving authentication, authorization, rate limiting, and monitoring functions. API gateways not only enhance system security and reliability but also simplify client-to-service interactions.
11. Service Discovery and Registration: Dynamic Service Management
Service discovery and registration tools are vital for understanding how microservices work by enabling dynamic registration and discovery of services, enhancing system elasticity and reliability. Common tools include:
- Consul: An open-source service mesh solution offering service discovery, configuration management, and health checks. Consul’s versatility and ease of use make it highly useful in building complex service meshes.
- Eureka: A service registry and discovery tool open-sourced by Netflix, widely used in the Spring Cloud ecosystem. Eureka’s simple configuration and powerful features make it highly efficient in microservices projects in the Spring environment.
- Zookeeper: An open-source distributed coordination service offering high availability and consistent distributed data management. Zookeeper’s reliability and powerful features make it highly effective in distributed systems requiring high consistency and reliability.
Using service discovery and registration, I can simplify service deployment and expansion, enhancing system elasticity and reliability. Service discovery and registration not only improve system flexibility but also simplify service management and maintenance.
12. Container Orchestration: Automated Management
Container orchestration tools are crucial for understanding how microservices work by providing automated deployment, scaling, and management of containerized applications, ensuring system high availability and scalability. Common orchestration tools include:
- Docker Swarm: Docker’s native orchestration tool, supporting simple cluster management and container scheduling. Docker Swarm’s simple configuration and efficient management make it useful in small clusters.
- Kubernetes: An open-source container orchestration platform offering automated deployment, scaling, and management features, and is the most popular container orchestration tool today. Kubernetes’s powerful features and broad support make it the top choice for building large-scale container clusters.
- HashiCorp: Offers multiple infrastructure automation tools like Terraform, Consul, Nomad, supporting container orchestration and service discovery. HashiCorp’s tool combination is highly useful in building complex automated infrastructures.
- OpenShift: An enterprise-grade container platform by Red Hat, built on Kubernetes, offering additional development and operations tools. OpenShift’s enterprise support and additional features make it highly effective in high-reliability enterprise environments.
Using container orchestration tools, I can achieve automated management of containers, ensuring application high availability and scalability, simplifying microservices operations. Container orchestration not only improves system management efficiency but also simplifies large-scale container cluster deployment and maintenance.
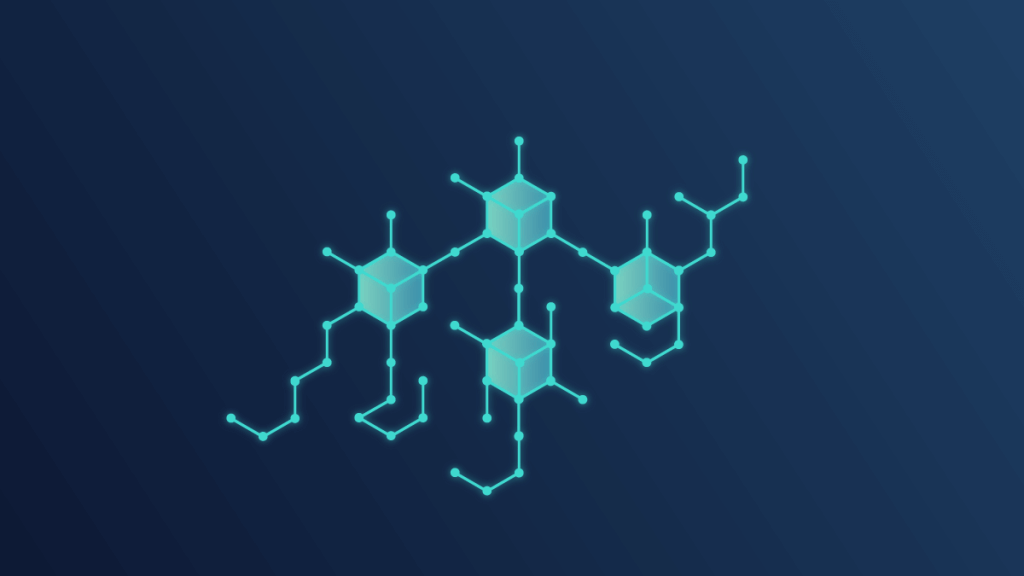
13. Distributed Tracing: End-to-End Monitoring
Distributed tracing tools are essential for understanding how microservices work by providing cross-service call chain tracing and analysis capabilities, helping developers quickly locate and resolve performance issues. Common tools include:
- OpenTelemetry: An open-source standard for distributed tracing and metrics, offering unified interfaces and tools, supporting multiple languages and frameworks. OpenTelemetry’s versatility and broad support make it highly useful in building distributed systems.
- Zipkin: An open-source distributed tracing system initially developed by Twitter, offering visualization and analysis of microservice call chains. Zipkin’s simple deployment and powerful features make it highly effective in systems needing quick performance diagnostics.
With distributed tracing, I can comprehensively understand the interactions between services, quickly identify performance bottlenecks and failure points, optimizing system reliability and performance. Distributed tracing not only improves system transparency but also helps development teams quickly locate and resolve issues, enhancing development efficiency.
14. API Management: Comprehensive API Lifecycle Management
API management tools are crucial for understanding how microservices work by providing comprehensive API lifecycle management features, including design, development, testing, release, and monitoring, ensuring high-quality and high-availability APIs. Common tools include:
- MuleSoft: An integration platform offering comprehensive API management and integration solutions, suitable for complex enterprise applications. MuleSoft’s powerful features and flexible integration capabilities make it highly effective in building and managing complex APIs.
- RunScope: An API testing and monitoring tool offering automated testing, performance monitoring, and failure diagnostics. RunScope’s ease of use and powerful features make it highly effective in systems needing high-quality API testing and monitoring.
Using API management tools, I can efficiently manage and maintain APIs, ensuring API security, reliability, and performance to meet evolving business needs. API management not only improves system stability but also ensures high-quality and high-availability APIs.
Final Thoughts
Understanding how microservices work involves breaking down a monolithic application into multiple independent services, achieving high availability, scalability, and flexibility. In this article, I shared some of my experiences and insights into microservices architecture, from the basics of container technology to advanced API management. I hope these insights provide you with a comprehensive guide to microservices architecture, helping you effectively apply these technologies and tools in your development projects. By understanding and mastering these technologies, we can build robust and efficient microservices systems to meet the ever-changing needs of modern enterprises.