Server-Side Rendering (SSR) represents a pivotal technique within the realm of modern web development. By allowing applications to render web pages on the server side rather than in the browser, SSR strikes a balance between dynamic interactivity and improved performance. This article delves into the concept of SSR, its advantages, and a detailed implementation example using React and Express.
Understanding SSR and its Advantages
In the traditional Client-Side Rendering (CSR) model, web applications rely on JavaScript to render content on the client’s browser. While CSR has brought about dynamic and interactive user experiences, it comes with its share of challenges. The initial load time can be extended due to the need to fetch and compile JavaScript libraries before rendering, impacting the perceived performance. Additionally, issues such as search engine optimization (SEO) and browser history management emerge as pain points.
SSR offers a solution by allowing the server to pre-render the web page before sending it to the client. This approach mitigates the slow initial load times associated with CSR. The server generates a fully-rendered HTML page, which is then enriched with client-side interactivity through JavaScript bundles. Consequently, the user is presented with a complete page right from the start, resulting in improved user experience and better SEO.
Implementation of SSR with React and Express
To better understand how SSR works, let’s explore a step-by-step implementation using React and Express:
- Project Initialization and Setup:
Begin by initializing a new React project using thecreate-react-app
command. This will serve as the foundation of our SSR implementation. Install the Express library as well, as it will be used to create the server that handles SSR. - Express Server Configuration:
Create an Express server in theserver/index.js
file. This server will be responsible for receiving requests, rendering React components on the server side, and sending the fully-rendered content to the client. - Server-Side Rendering Function:
Develop a server-rendering function, such asserverRenderer.js
, that orchestrates the SSR process. This function reads an HTML template, renders the React component into HTML usingReactDOMServer.renderToString()
, and inserts the HTML content into the template. - ESM to CommonJS Transformation:
For running the server code with Node.js, you’ll need to convert the ECMAScript Modules (ESM) syntax to CommonJS. This can be achieved using tools like Babel and packages likeignore-styles
. - Building and Starting the Server:
Once the implementation is complete, build the React project and start the SSR server. The server will now render the React component on the server side, generating fully-rendered HTML pages to be delivered to clients.
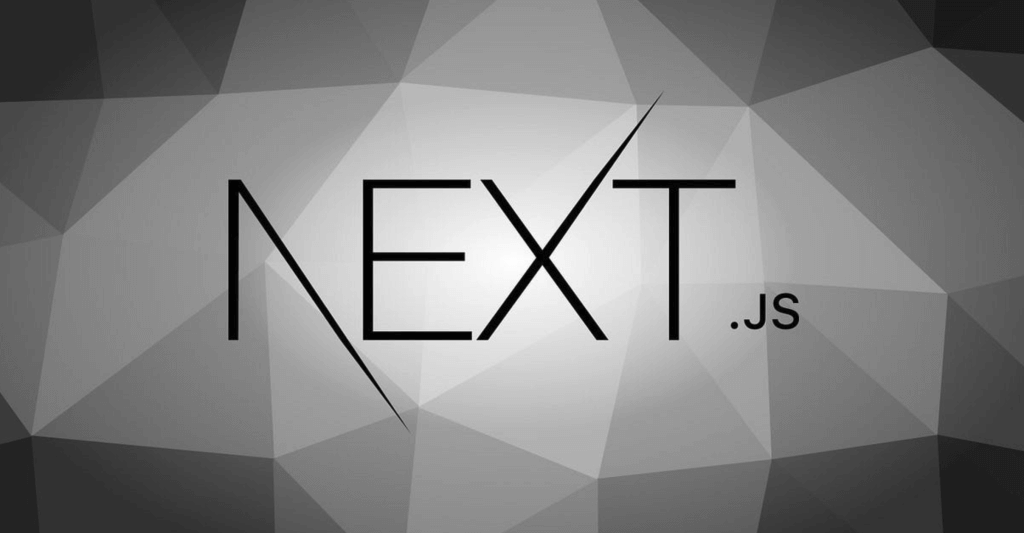
Comparing SSR with CSR and Next.js
It’s important to distinguish between SSR and Client-Side Rendering (CSR). While SSR involves pre-rendering content on the server and delivering complete HTML pages, CSR focuses on rendering content in the browser using JavaScript. Next.js, a popular framework for React, offers a streamlined way to implement SSR. It abstracts away much of the complex configuration and provides built-in SSR capabilities, making the process smoother and more efficient.
Conclusion
Server-Side Rendering (SSR) stands as a potent solution to the challenges posed by traditional Client-Side Rendering (CSR). By rendering web pages on the server side and sending fully-rendered HTML to the client, SSR enhances initial load times, SEO, and user experience. The detailed implementation example using React and Express provides insights into the technical aspects of SSR. As modern web development continues to evolve, understanding SSR’s capabilities and advantages becomes increasingly relevant for creating performant and user-friendly applications.