In the realm of computer software system development, efficiently handling concurrent I/O is a timeless topic. I once faced a challenge in a project where I needed to design a high-performance server capable of handling thousands of simultaneous network connections. Through this process, I delved into the applications of the Reactor model and epoll. These technologies ultimately helped me solve the problem. Today, I would like to share my exploration and experiences in this area.
Discovering the Reactor Model
One summer afternoon, I noticed that the server’s performance significantly dropped when the number of concurrent connections was high. Traditional methods like select and poll were clearly inadequate. So, I began to study the Reactor model.
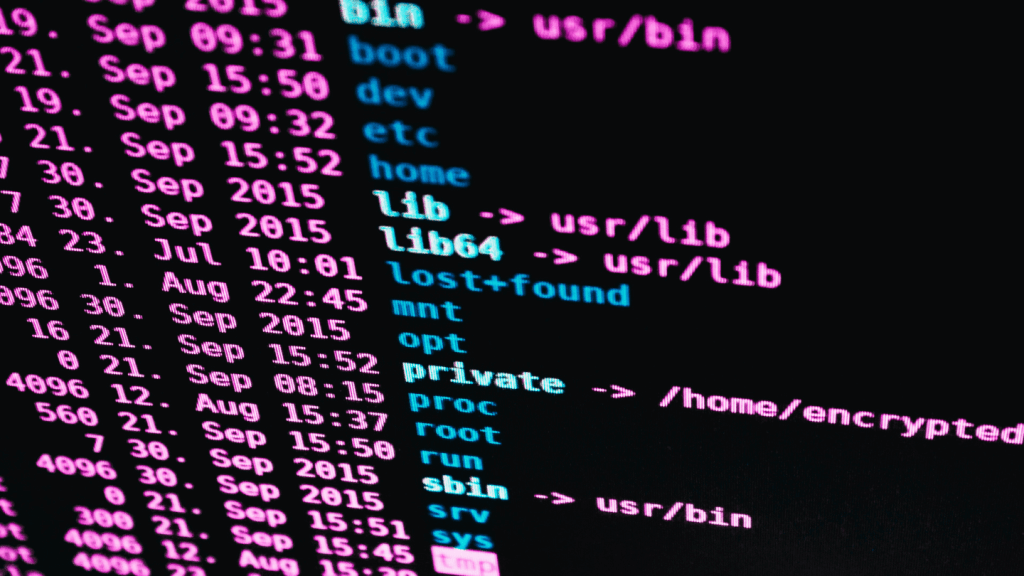
The Reactor model is a design pattern used for handling concurrent I/O operations. Its core idea is to separate I/O operations from business logic through an event-driven mechanism. The model comprises three main components: the multiplexer, the event dispatcher, and the event handler.
- Multiplexer: Monitors multiple file descriptors to detect which ones have events. In Linux, the multiplexer is usually implemented with select, poll, or epoll.
- Event Dispatcher: After receiving events from the multiplexer, it dispatches them to the corresponding event handlers.
- Event Handler: Responsible for handling specific I/O events, such as reading data and processing requests.
In the Reactor model, all I/O operations are asynchronous, and the application is notified to process events only when they occur. This greatly enhances the system’s ability to handle concurrent operations.
Applying epoll
After understanding the basic concepts of the Reactor model, I further explored epoll. epoll is a high-performance I/O multiplexing mechanism provided by the Linux kernel, designed specifically for handling large-scale concurrent connections.
The advantage of epoll lies in its event-driven mechanism and efficient event notification. Compared to select and poll, epoll offers significant improvements in performance and scalability, especially when dealing with a large number of file descriptors. To better understand and apply this technology, I wrote a simple epoll example:
#include <sys/epoll.h>
#include <fcntl.h>
#include <unistd.h>
#include <stdio.h>
#define MAX_EVENTS 10
int main() {
int epoll_fd = epoll_create1(0);
if (epoll_fd == -1) {
perror("epoll_create1");
return 1;
}
int listen_fd = open("somefile", O_RDONLY);
if (listen_fd == -1) {
perror("open");
return 1;
}
struct epoll_event event;
event.events = EPOLLIN;
event.data.fd = listen_fd;
if (epoll_ctl(epoll_fd, EPOLL_CTL_ADD, listen_fd, &event) == -1) {
perror("epoll_ctl");
return 1;
}
struct epoll_event events[MAX_EVENTS];
int n;
while (1) {
n = epoll_wait(epoll_fd, events, MAX_EVENTS, -1);
for (int i = 0; i < n; i++) {
if (events[i].events & EPOLLIN) {
// Handle readable events
char buffer[128];
read(events[i].data.fd, buffer, sizeof(buffer));
printf("Read data: %s\n", buffer);
}
}
}
close(listen_fd);
close(epoll_fd);
return 0;
}
This example demonstrates how to create an epoll instance, add file descriptors to it, and handle events when they occur. Through this approach, I was able to efficiently manage the concurrent connections on the server, significantly improving the system’s performance.
Reflections and Conclusions from Practice
Through this exploration, I not only resolved the server performance issues but also gained a deeper understanding of the Reactor model and epoll. It became clear that the secret to high-performance servers lies in event-driven and asynchronous I/O. This realization highlighted the relentless pursuit of efficiency that drives technological advancement.
The combination of the Reactor model and epoll provides powerful tools for handling high-concurrency I/O. These technologies are not only suitable for server development but are also widely used in various scenarios requiring efficient I/O processing. I hope this article helps more developers understand and apply these techniques to enhance system performance and scalability.
Whether you are a novice or an experienced developer, when faced with the challenge of concurrent I/O processing, consider trying the Reactor model and epoll. You might discover a whole new world.