At a blockchain developer conference, I encountered a novice smart contract developer named Alex. With a puzzled expression, he asked me, “Why is my transaction on Ethereum stuck in a pending state?” This question not only perplexed Alex but also many blockchain enthusiasts and developers. Today, I’d like to take this opportunity to share insights on the issue of long-pending transactions.
Alex’s Dilemma
Alex, a young developer passionate about blockchain technology, had recently deployed his first smart contract. When he attempted a simple transfer operation, the transaction was delayed indefinitely, remaining in a pending state. This frustrated him because he couldn’t understand why this was happening or how to resolve it.
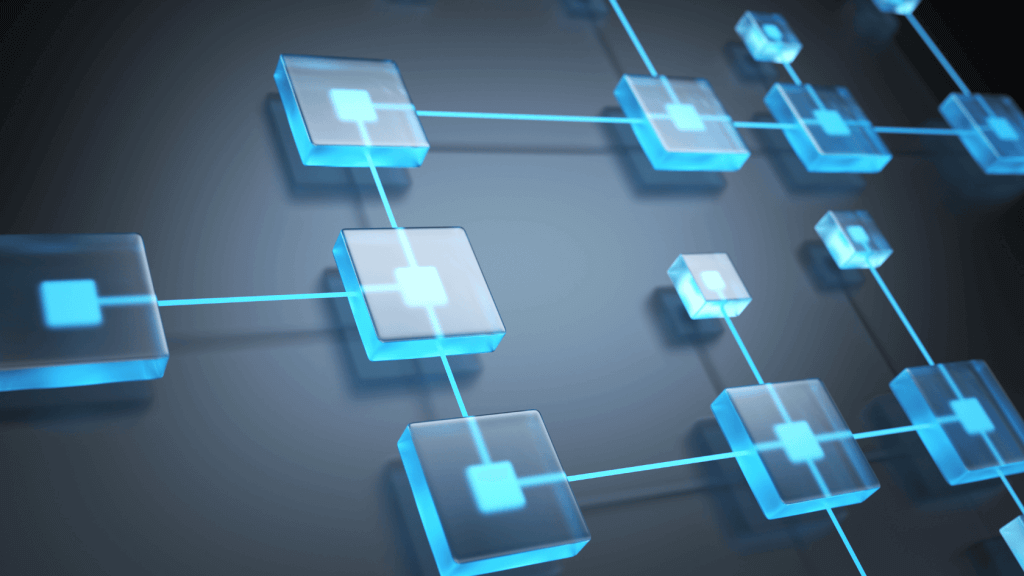
The Journey of a Transaction
Every transaction embarks on a unique journey from initiation to final confirmation. Initially, when a user sends a transaction, it is broadcasted to various network nodes and temporarily stored in the memory pool (mempool). Miners then select transactions from the mempool to include in blocks for confirmation. However, not all transactions are prioritized, much like waiting in an airport security line where some people get fast-track passes while others wait.
Factors Affecting Transaction Confirmation
1. Gas Fee Amount:
Every transaction on the Ethereum network requires a gas fee. Miners tend to prioritize transactions with higher gas fees because it increases their earnings. If Alex set a low gas fee, miners might prioritize other transactions with higher rewards, leaving his transaction in a pending state.
// Example of setting a gas fee in a Go Ethereum client
import (
"github.com/ethereum/go-ethereum/common"
"github.com/ethereum/go-ethereum/core/types"
"github.com/ethereum/go-ethereum/ethclient"
"math/big"
"context"
)
func sendTransaction(client *ethclient.Client, privateKey string, toAddress string, value *big.Int, gasLimit uint64, gasPrice *big.Int) (common.Hash, error) {
fromAddress := // derive from privateKey
nonce, err := client.PendingNonceAt(context.Background(), fromAddress)
if err != nil {
return common.Hash{}, err
}
tx := types.NewTransaction(nonce, common.HexToAddress(toAddress), value, gasLimit, gasPrice, nil)
// Sign and send the transaction
}
2. Network Congestion:
The blockchain network isn’t always smooth and uninterrupted; it can experience congestion. During peak times, transaction volumes surge, causing mempool transactions to pile up. In such instances, Alex’s transaction confirmation time could be significantly prolonged.
// Function to check network congestion
func checkPendingTransactions(client *ethclient.Client) (int, error) {
pendingTxCount, err := client.PendingTransactionCount(context.Background())
if err != nil {
return 0, err
}
return int(pendingTxCount), nil
}
3. Transaction Sequence:
Transactions on Ethereum are processed in nonce order. If Alex has an unconfirmed previous transaction, subsequent transactions will also be blocked in a pending state until the earlier one is processed.
// Example of handling nonce
nonce, err := client.PendingNonceAt(context.Background(), fromAddress)
if err != nil {
log.Fatal(err)
}
// Ensure the nonce is correct for the new transaction
tx := types.NewTransaction(nonce, common.HexToAddress(toAddress), value, gasLimit, gasPrice, nil)
How to Handle Long-Pending Transactions
1. Increase Gas Fee:
Alex could try rebroadcasting the transaction with a higher gas fee. This increases the likelihood of the transaction being picked by miners. Many wallets and transaction tools now support the “replace-by-fee” strategy, allowing users to pay higher fees to expedite transaction confirmation.
// Example of replacing a transaction with higher gas fee
newGasPrice := big.NewInt(2).Mul(gasPrice, big.NewInt(2)) // Double the gas price
tx := types.NewTransaction(nonce, common.HexToAddress(toAddress), value, gasLimit, newGasPrice, nil)
2. Cancel the Transaction:
If the transaction remains unconfirmed, Alex could attempt to send a null transaction with the same nonce (sending 0 ETH to himself) to cancel the pending transaction. This method can clear the mempool of stuck transactions, allowing subsequent ones to proceed smoothly.
// Example of canceling a transaction by sending a 0 ETH transaction
tx := types.NewTransaction(nonce, fromAddress, big.NewInt(0), gasLimit, gasPrice, nil)
3. Be Patient:
Sometimes, the best solution is to wait. Although a pending transaction can cause anxiety, patience can be a virtue during network congestion. Most transactions will eventually be confirmed; it’s just a matter of time.
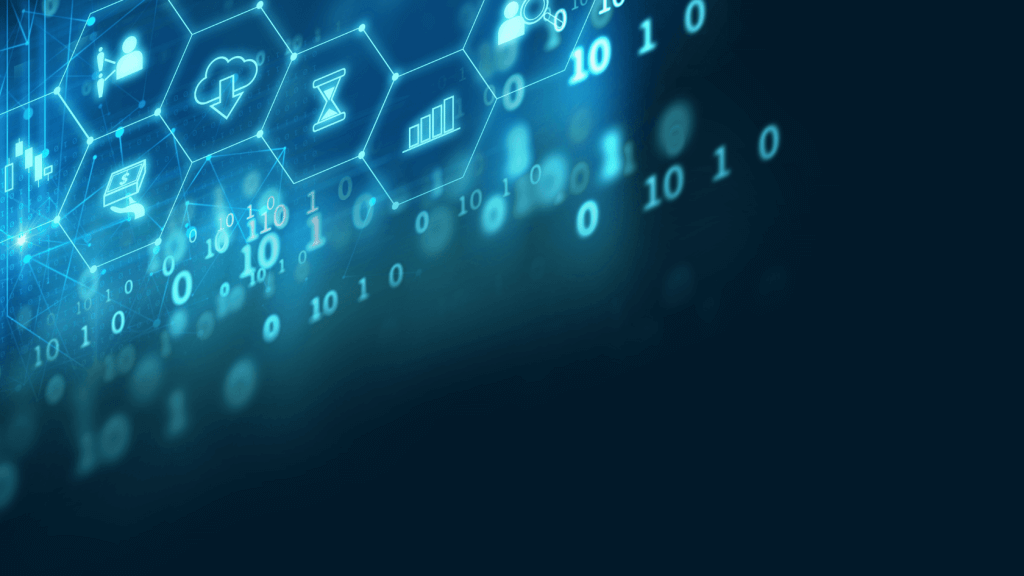
Personal Experience and Insights
Reflecting on my early days with blockchain technology, I too faced dilemmas similar to Alex’s. When I deployed a complex smart contract on Ethereum for the first time, I encountered a pending transaction issue. At that time, I was at a loss and didn’t know how to resolve it. Through continuous learning and experimentation, I gradually mastered various methods to handle pending transactions and deeply understood the underlying mechanisms of the blockchain network.
Through this interaction with Alex at the conference, I not only helped him solve his problem but also once again appreciated the complexity and charm of blockchain technology. Each pending transaction reflects the profound intricacies of the underlying technology and the challenges of real-world applications. As developers, we must constantly learn, improve our technical skills, and maintain patience and composure to handle unforeseen issues.
I hope this sharing can help more developers like Alex resolve the confusion of pending transactions, allowing everyone to better explore and progress in the world of blockchain. Remember, every challenge is an opportunity for learning and growth. Let’s embrace the future of blockchain technology together and explore its vast potential.